ASP.NET Web APIs Skill Overview
Welcome to the ASP.NET Web APIs Skill page. You can use this skill
template as is or customize it to fit your needs and environment.
- Category: Technical > API
Description
ASP.NET Web APIs are a framework for building HTTP-based services that can be accessed from various clients, including browsers and mobile devices. They enable developers to create RESTful applications, which use standard HTTP methods like GET, POST, PUT, and DELETE to perform CRUD operations. With ASP.NET Web APIs, you can easily expose data and functionality over the web, allowing seamless integration with other applications and services. The framework supports features such as routing, model binding, validation, and security, making it a robust choice for developing scalable and maintainable web services. It is particularly useful for creating APIs that serve as the backend for single-page applications or mobile apps.
Expected Behaviors
Micro Skills
Explaining the role of Web APIs in modern web applications
Identifying key components of ASP.NET Web API architecture
Describing the client-server model in the context of Web APIs
Recognizing the benefits of using ASP.NET for building Web APIs
Defining the purpose of each HTTP method
Demonstrating how to use HTTP methods in API requests
Understanding idempotency and its relevance to HTTP methods
Explaining the difference between safe and unsafe HTTP methods
Defining REST and its core principles
Understanding resource representation in RESTful APIs
Explaining statelessness in RESTful services
Identifying best practices for designing RESTful APIs
Installing and setting up Visual Studio for ASP.NET development
Selecting the appropriate project template for Web API
Configuring project settings such as target framework and authentication
Building and running the initial project to verify setup
Understanding the structure of a controller in ASP.NET Web APIs
Creating a new controller class and defining action methods
Mapping HTTP methods to action methods using attributes
Returning data from action methods using IActionResult
Understanding the default routing conventions in ASP.NET Web APIs
Customizing routes using attribute routing
Defining route parameters and constraints
Testing routes to ensure correct endpoint mapping
Reading data from HTTP request bodies
Sending data in HTTP response bodies
Setting HTTP status codes in responses
Using content negotiation to return different data formats
Defining DTO classes to encapsulate data for API requests and responses
Mapping domain models to DTOs using libraries like AutoMapper
Ensuring DTOs are serializable for JSON/XML formats
Validating DTOs to ensure data integrity before processing
Understanding the model binding process in ASP.NET Web APIs
Configuring custom model binders for complex types
Implementing data annotations for model validation
Handling model state errors in API responses
Configuring authentication schemes such as Basic, Bearer, or OAuth
Implementing role-based access control (RBAC) for API endpoints
Using ASP.NET Identity for user management and authentication
Protecting sensitive data with HTTPS and secure headers
Configuring global exception handling using middleware
Creating custom exception filters for specific error scenarios
Logging API activity and errors using frameworks like Serilog
Returning standardized error responses to clients
Implementing server-side caching using memory cache
Configuring client-side caching with cache-control headers
Utilizing response compression to reduce payload size
Analyzing API performance using profiling tools
Understanding different versioning techniques (URL, query string, header)
Configuring URL-based versioning in ASP.NET Web APIs
Managing backward compatibility with deprecated endpoints
Documenting API versions for consumer clarity
Using NuGet packages to add functionality to Web APIs
Configuring and consuming external RESTful services
Handling asynchronous operations with third-party services
Ensuring security and data integrity when integrating external services
Creating and running test collections in Postman
Automating API tests with Postman scripts
Generating and customizing API documentation with Swagger
Debugging API requests and responses using built-in browser tools
Analyzing business requirements to determine API design
Applying SOLID principles to API architecture
Utilizing microservices architecture for scalability
Implementing API gateways for managing multiple APIs
Designing for horizontal scaling and load balancing
Configuring OAuth 2.0 authentication flows
Implementing JWT token generation and validation
Securing API endpoints with role-based access control
Integrating identity providers for single sign-on
Applying best practices for storing and transmitting tokens
Configuring dependency injection containers
Registering services and interfaces for DI
Creating custom middleware components
Managing request pipelines with middleware
Optimizing middleware for performance and security
Establishing code review guidelines and checklists
Identifying and resolving code smells and anti-patterns
Using profiling tools to analyze API performance
Conducting load testing to assess API scalability
Documenting findings and recommendations for improvement
Tech Experts
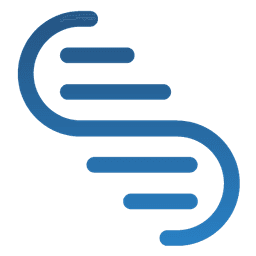
StackFactor Team
We pride ourselves on utilizing a team of seasoned experts who diligently curate roles, skills, and learning paths by harnessing the power of artificial intelligence and conducting extensive research. Our cutting-edge approach ensures that we not only identify the most relevant opportunities for growth and development but also tailor them to the unique needs and aspirations of each individual. This synergy between human expertise and advanced technology allows us to deliver an exceptional, personalized experience that empowers everybody to thrive in their professional journeys.