Angular Reactive Extensions (NgRx) Skill Overview
Welcome to the Angular Reactive Extensions (NgRx) Skill page. You can use this skill
template as is or customize it to fit your needs and environment.
- Category: Technical > Programming frameworks
Description
Angular Reactive Extensions, or NgRx, is a framework for managing state and side effects in Angular applications. It uses the Redux pattern with RxJS to provide a predictable state container, making it easier to track changes and handle complex scenarios. In NgRx, you dispatch actions that are handled by reducers to update the state. Side effects, like data fetching, are managed separately using Effects. This separation of concerns makes your code more maintainable and easier to test. NgRx also integrates well with Angular's component architecture, allowing you to select slices of state and reactively display them in your components.
Expected Behaviors
Micro Skills
Knowing what is state management
Understanding how NgRx fits into state management
Recognizing the core principles of NgRx
Identifying the problems that NgRx solves
Comparing NgRx with other state management solutions
Understanding the performance benefits of using NgRx
Defining what an Action is in NgRx
Defining what a Reducer is in NgRx
Defining what an Effect is in NgRx
Understanding how NgRx integrates with Angular
Knowing when to use NgRx in an Angular application
Recognizing the impact of NgRx on application architecture
Understanding which packages are needed for NgRx
Using npm or yarn to install packages
Importing StoreModule into AppModule
Calling StoreModule.forRoot in imports array
Understanding what an interface is in TypeScript
Deciding what properties the state should have
Knowing how to define a constant in TypeScript
Assigning initial values to state properties
Understanding the purpose of forRoot method
Passing the initial state and reducer to forRoot
Choosing descriptive names for action types
Understanding the purpose of action creators
Using createAction function to create action creators
Injecting the store into a component or service
Calling store.dispatch with an action
Understanding the reducer function signature
Using the on function to handle specific actions
Knowing the order of actions, reducers, and selectors in data flow
Understanding how components react to state changes
Handling multiple action types in a reducer
Using the on function to handle actions
Working with nested state objects
Updating state immutably
Creating basic selectors with the createSelector function
Composing selectors for complex queries
Using selectors with props
Memoization in selectors
Creating an Effect that listens for an action
Mapping an action to a different action in an Effect
Handling errors in Effects
Using non-dispatching Effects
Registering multiple stores in the AppModule
Dispatching actions to specific stores
Selecting data from specific stores
Understanding when to use multiple stores vs. a single store with nested state
Installing and setting up Redux Devtools
Inspecting the current state of the application
Viewing dispatched actions and their payloads
Time-travel debugging
Understanding the concept of entity adapters
Creating an entity adapter
Using entity adapter methods to manipulate state
Optimizing selectors with entity adapters
Understanding the role of Effects in handling side effects
Creating complex Effects using RxJS operators
Handling errors within Effects
Testing Effects
Understanding testing strategies for NgRx
Writing unit tests for Actions, Reducers, and Selectors
Writing integration tests for Effects
Mocking store data for testing
Understanding the facade pattern
Creating a facade service
Using facades to simplify component interaction with the store
Testing facades
Understanding how to integrate NgRx with Angular Router
Integrating NgRx with third-party APIs
Using NgRx with other libraries like Angular Material
Handling authentication and authorization with NgRx
Understanding the requirements of large-scale applications
Planning the state structure for scalability and maintainability
Deciding on the appropriate use of actions, reducers, selectors, and effects
Designing the interaction between different stores
Ensuring the application architecture adheres to best practices
Profiling and identifying performance bottlenecks
Optimizing the use of selectors
Implementing lazy loading of stores
Reducing action dispatch overhead
Optimizing the use of Effects for asynchronous operations
Using advanced features of Redux Devtools
Debugging complex scenarios involving multiple actions and effects
Identifying and fixing issues related to state mutation
Troubleshooting performance issues
Understanding and resolving common pitfalls and anti-patterns in NgRx
Understanding the codebase and architecture of NgRx
Identifying areas of improvement or new feature possibilities
Writing high-quality, testable code
Following the contribution guidelines and code of conduct
Collaborating effectively with the NgRx team and community
Explaining complex concepts in a simple, understandable way
Creating learning materials like tutorials, blog posts, and videos
Providing constructive feedback and guidance
Staying updated with the latest changes in NgRx
Promoting best practices and encouraging continuous learning
Tech Experts
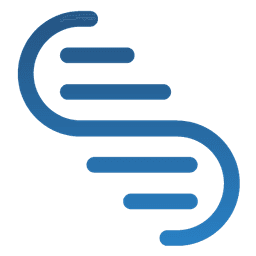
StackFactor Team
We pride ourselves on utilizing a team of seasoned experts who diligently curate roles, skills, and learning paths by harnessing the power of artificial intelligence and conducting extensive research. Our cutting-edge approach ensures that we not only identify the most relevant opportunities for growth and development but also tailor them to the unique needs and aspirations of each individual. This synergy between human expertise and advanced technology allows us to deliver an exceptional, personalized experience that empowers everybody to thrive in their professional journeys.