.NET Core Skill Overview
Welcome to the .NET Core Skill page. You can use this skill
template as is or customize it to fit your needs and environment.
- Category: Technical > Programming frameworks
Description
.NET Core is a versatile, open-source framework developed by Microsoft for building modern applications. It supports C# programming and allows developers to create web applications, services, and even IoT apps across multiple platforms like Windows, Linux, and macOS. .NET Core includes ASP.NET Core for web development and Entity Framework Core for database operations. It uses the MVC (Model-View-Controller) architecture, enabling clean code organization. Other features include dependency injection for better code maintainability, LINQ for data manipulation, and SignalR for real-time web functionality. Advanced users can optimize performance, debug complex issues, and even contribute to its open-source project.
Stack
.NET,
Expected Behaviors
Micro Skills
Familiarity with the purpose and benefits of .NET Core
Basic knowledge of .NET Core's cross-platform capabilities
Understanding of the .NET Core command-line interface (CLI)
Awareness of the .NET Core project structure
Understanding of basic C# data types
Knowledge of control flow structures in C#
Ability to declare and use variables in C#
Understanding of basic object-oriented programming concepts in C#
Understanding of what ASP.NET Core is used for
Familiarity with the basic components of an ASP.NET Core application
Awareness of how to create a simple ASP.NET Core web application
Basic understanding of how to handle requests and responses in ASP.NET Core
Familiarity with the concept of Model-View-Controller (MVC)
Understanding of how MVC is implemented in .NET Core
Basic knowledge of how to create models, views, and controllers in .NET Core
Awareness of the role of routing in MVC applications
Understanding of what Entity Framework Core is and what it's used for
Familiarity with the concept of Object-Relational Mapping (ORM)
Basic knowledge of how to use Entity Framework Core to interact with a database
Awareness of how to define models in Entity Framework Core
Knowledge of the purpose of project files
Understanding of the organization of code files
Ability to create a new project using the CLI
Understanding of how to build and run a project using the CLI
Ability to manage NuGet packages using the CLI
Understanding of C# syntax
Ability to write simple functions
Ability to start an application in debug mode
Understanding of how to inspect variables during debugging
Knowledge of Select operator
Understanding of Where operator
Ability to use OrderBy operator
Familiarity with GroupBy operator
Understanding of Join operations in LINQ
Knowledge of how to use Aggregate functions
Ability to use nested queries
Understanding of how to use the Let keyword
Understanding of how to write LINQ queries against a DbContext
Knowledge of how to use Include and ThenInclude for eager loading
Ability to handle transactions using LINQ
Understanding of how to use LINQ projections
Knowledge of when a query is executed
Understanding of how to force immediate execution
Ability to use deferred execution to improve performance
Knowledge of HTTP methods
Understanding of status codes
Familiarity with the concept of statelessness
Awareness of resource identification
Understanding of how to send GET requests
Ability to send POST requests
Knowledge of how to handle response status codes
Understanding of how to deserialize JSON responses
Understanding of how to define routes
Knowledge of how to create action methods
Ability to return appropriate status codes
Understanding of how to model bind parameters
Knowledge of how to use authentication middleware
Understanding of how to implement authorization policies
Ability to use HTTPS for secure communication
Knowledge of how to protect against CSRF attacks
Understanding of how to declare asynchronous methods
Ability to call asynchronous methods
Knowledge of how to use Task and Task<T>
Understanding of how to avoid deadlocks
Knowledge of how to start a new Task
Ability to use Task.Run for CPU-bound operations
Understanding of how to cancel tasks
Knowledge of how to handle exceptions in tasks
Understanding of how exceptions propagate in async code
Knowledge of how to use try/catch blocks with async/await
Ability to use Task.Exception property
Understanding of IAsyncEnumerable interface
Ability to use await foreach loop
Knowledge of how to create an async stream
Knowledge of how to configure Identity options
Ability to manage users and roles
Understanding of how to use sign-in manager
Knowledge of how to customize Identity UI
Understanding of how to configure cookie options
Ability to issue and validate cookies
Knowledge of how to handle sign-out
Understanding of how to protect against cross-site request forgery (CSRF)
Understanding of how to generate JWTs
Ability to validate JWTs
Knowledge of how to handle token expiration
Understanding of how to secure JWTs
Knowledge of how to assign roles to users
Ability to check roles in controllers and views
Understanding of how to define policies
Knowledge of how to enforce policies
Knowledge of how to use Razor expressions
Ability to use Razor control structures
Understanding of how to use Razor HTML helpers
Knowledge of how to create Razor components
Understanding of how to define a page model
Ability to handle GET and POST requests
Knowledge of how to use ViewData and ViewBag
Understanding of how to use model binding
Understanding of how to create form controls
Ability to validate form data
Knowledge of how to handle file uploads
Understanding of how to prevent cross-site request forgery (CSRF)
Knowledge of how to use built-in Tag Helpers
Ability to create custom Tag Helpers
Understanding of how to use Tag Helper attributes
Knowledge of how to use Tag Helper methods
Knowledge of what shadow properties are
Ability to add a shadow property to an entity
Understanding of how to use shadow properties in queries
Knowledge of how to update shadow properties
Understanding of one-to-one, one-to-many, and many-to-many relationships
Ability to configure relationships using Fluent API
Knowledge of how to use navigation properties
Understanding of how to handle loading related data
Understanding of how to configure entity properties
Knowledge of how to configure relationships
Ability to configure inheritance hierarchies
Understanding of how to override conventions
Knowledge of how to create a new migration
Ability to apply and rollback migrations
Understanding of how to customize a migration
Knowledge of how to handle model changes that require complex migrations
Understanding of what concurrency conflicts are
Ability to detect concurrency conflicts
Knowledge of how to resolve concurrency conflicts
Understanding of how to test for concurrency conflicts
Knowledge of what microservices are
Understanding of the benefits and challenges of microservices
Knowledge of common microservice patterns
Understanding of how to design a microservice architecture
Understanding of how to define a service boundary
Ability to design a microservice API
Knowledge of how to implement a microservice using .NET Core
Understanding of how to test a microservice
Understanding of what Docker is
Knowledge of how to create a Dockerfile
Ability to build and run a Docker image
Understanding of how to use Docker Compose
Knowledge of synchronous vs. asynchronous communication
Ability to use HTTP/REST for synchronous communication
Understanding of how to use message queues for asynchronous communication
Knowledge of how to handle errors and retries in communication
Understanding of database per service pattern
Knowledge of how to handle transactions across services
Ability to implement data synchronization between services
Understanding of how to handle querying across services
Knowledge of CLR (Common Language Runtime)
Understanding of .NET Core's Base Class Library
Knowledge of .NET Core's Intermediate Language (IL)
Proficiency in using .NET Core's performance profiling tools
Understanding of how to optimize .NET Core's memory usage
Ability to optimize .NET Core's CPU usage
Ability to use advanced debugging tools like WinDbg
Understanding of how to debug .NET Core's memory leaks
Ability to debug .NET Core's threading issues
Understanding of how to configure .NET Core's garbage collector
Ability to modify .NET Core's threading behavior
Understanding of how to change .NET Core's security settings
Understanding of the .NET Core project's contribution guidelines
Ability to write high-quality code that adheres to the .NET Core project's standards
Knowledge of how to submit a pull request to the .NET Core project
Tech Experts
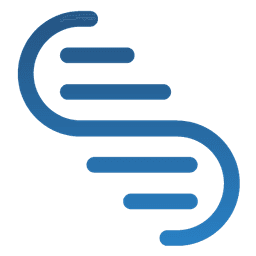
StackFactor Team
We pride ourselves on utilizing a team of seasoned experts who diligently curate roles, skills, and learning paths by harnessing the power of artificial intelligence and conducting extensive research. Our cutting-edge approach ensures that we not only identify the most relevant opportunities for growth and development but also tailor them to the unique needs and aspirations of each individual. This synergy between human expertise and advanced technology allows us to deliver an exceptional, personalized experience that empowers everybody to thrive in their professional journeys.